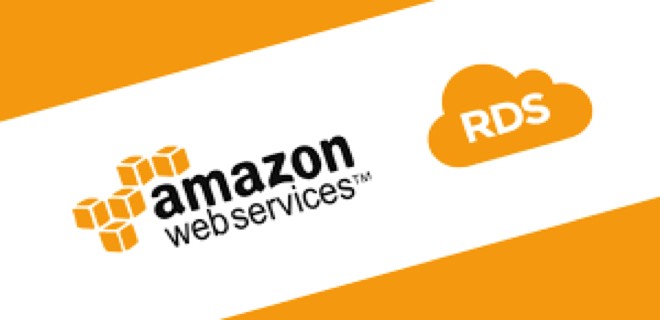
Добавление RDS хостов в zabbix c использованием boto3 + python3
На работе потребовалось добавлять RDS хосты (с различных учеток AWS) в заббикс. Руками делать, — это конечно нормальное явление, но я люблю оптимизацию и автоматизацию. И для этого готов был написать скрипт. Пал выбор на питон.
Полезное чтиво:
Установка Zabbix + nginx+php-fpm + mariaDB в Unix/Linux
Установка Zabbix-agent в Unix/Linux
Установка pip/setuptools/wheel в Unix/Linux
Автоматическое выключение хостов в zabbix
Автоматическое удаление хостов в zabbix
Открываем файл:
# vim Add_Hosts_from_AWS_RDS_to_Zabbix.py
Он выглядит следующим образом:
#!/bin/env python3 # -*- coding: utf-8 -*- import requests import sys import boto3 import glob import smtplib from pyzabbix import ZabbixAPI, ZabbixAPIException # class bgcolors: def __init__(self): self.colors = { 'HEADER': '\033[95m', 'OKBLUE': '\033[94m', 'OKGREEN': '\033[92m', 'WARNING': '\033[93m', 'FAIL': '\033[91m', 'ENDC': '\033[0m', 'BOLD': '\033[1m', 'UNDERLINE': '\033[4m' } def get_creds_from_assum_role(): auth_params = [] # create an STS client object that represents a live connection to the # STS service #sts_client = boto3.setup_default_session(region_name='us-east-1') sts_client = boto3.client('sts', region_name='us-east-1') # Assum_Roles = ['arn:aws:iam::93388403184:role/CW-RDS-READ-ONLY' , 'arn:aws:iam::4131648289989:role/CLOUDWATCH-READONLY-ACCESS-CMSHARED' , 'arn:aws:iam::067785372661:role/CLOUDWATCH-READONLY-ACCESS-DEV' , 'arn:aws:iam::66803035257:role/CLOUDWATCH-READONLY-ACCESS-CMSHARED' , 'arn:aws:iam::505163668321:role/CLOUDWATCH-READONLY-ACCESS-ZABBIX' , 'arn:aws:iam::378494444851:role/CLOUDWATCH-READONLY-ACCESS-ZABBIX'] # Call the assume_role method of the STSConnection object and pass the role # ARN and a role session name. for Assum_Role in Assum_Roles: assumedRoleObject = sts_client.assume_role( RoleArn=Assum_Role, RoleSessionName="ElastiCache" ) # From the response that contains the assumed role, get the temporary # credentials that can be used to make subsequent API calls credentials = assumedRoleObject['Credentials'] auth_param = { 'access_key': credentials['AccessKeyId'], 'secret_key': credentials['SecretAccessKey'], 'session_token': credentials['SessionToken'] } auth_params.append(auth_param) # print(auth_params) return auth_params def get_rds_hosts_from_assum_role(): hosts_from_rds = [] # RDS Creds_Data = get_creds_from_assum_role() for get_greds_from_acc in Creds_Data: access_key = get_greds_from_acc['access_key'] secret_key = get_greds_from_acc['secret_key'] session_token = get_greds_from_acc['session_token'] # rds_client = boto3.setup_default_session(region_name='us-east-1' , aws_access_key_id=access_key , aws_secret_access_key=secret_key , aws_session_token=session_token) rds_client = boto3.client('rds' , region_name='us-east-1' , aws_access_key_id=access_key , aws_secret_access_key=secret_key , aws_session_token=session_token) try: # get all of the db instances rdses = rds_client.describe_db_instances() for rds in rdses['DBInstances']: hosts_from_rds.append(rds['Endpoint']['Address']) except Exception as error: print (error) # print(hosts_from_rds) return hosts_from_rds # def get_elasticache_hosts_from_assum_role(): def login_to_zabbix(): # host, login, password to connect to DEV zabbix-server Zabbix_Host = 'https://zabbix.com/' Zabbix_User = 'apiuser' Zabbix_Password = 'gkjhjhvjh5uigkjhg' # You can use the Connection_Timeout Connection_Timeout = 25 # Verify SSL Verify_SSL = False # Connect to zabbix-server zapi = ZabbixAPI(Zabbix_Host, timeout=Connection_Timeout) zapi.session.verify = Verify_SSL if not Verify_SSL: from requests.packages.urllib3.exceptions import InsecureRequestWarning requests.packages.urllib3.disable_warnings(InsecureRequestWarning) r = requests.get(Zabbix_Host, verify=Verify_SSL) # zapi.login(Zabbix_User, Zabbix_Password) zapi.session.auth = (Zabbix_User, Zabbix_Password) # You can re-define Connection_Timeout after zapi.timeout = Connection_Timeout return zapi def add_group_to_zabbix(group): zapi = login_to_zabbix() zapi.hostgroup.create(name=group) return add_group_to_zabbix def add_host_to_zabbix(host, group, template, port=666): zapi = login_to_zabbix() Get_Templates = zapi.template.get(search={"name": template}) for template in Get_Templates: # print(template['templateid']) templateid = template['templateid'] Get_Groups = zapi.hostgroup.get(search={"name": group}) for group in Get_Groups: if len(host) > 64: print ('Host is more than 64 characters: ', host) sender = 'captain@localhost' receivers = 'vitalii_natarov@epam.com' message = ('Host is more than 64 characters: %s' % host) smtpObj = smtplib.SMTP('localhost') smtpObj.sendmail(sender, receivers, message) else: zapi.host.create({"host": host, "interfaces": [{ "type": 1, "main": 1, "useip": 0, "ip": "", "dns": host, "port": port, }], "groups": [{ "groupid": group['groupid'], }], "templates": [{ "templateid": templateid, }], }) return add_host_to_zabbix def add_template_to_zabbix(): zapi = login_to_zabbix() rules = { 'applications': { 'createMissing': 'true', 'updateExisting': 'true' }, 'discoveryRules': { 'createMissing': 'true', 'updateExisting': 'true' }, 'graphs': { 'createMissing': 'true', 'updateExisting': 'true' }, 'groups': { 'createMissing': 'true' }, 'hosts': { 'createMissing': 'true', 'updateExisting': 'true' }, 'images': { 'createMissing': 'true', 'updateExisting': 'true' }, 'items': { 'createMissing': 'true', 'updateExisting': 'true' }, 'maps': { 'createMissing': 'true', 'updateExisting': 'true' }, 'screens': { 'createMissing': 'true', 'updateExisting': 'true' }, 'templateLinkage': { 'createMissing': 'true', 'updateExisting': 'true' }, 'templates': { 'createMissing': 'true', 'updateExisting': 'true' }, 'templateScreens': { 'createMissing': 'true', 'updateExisting': 'true' }, 'triggers': { 'createMissing': 'true', 'updateExisting': 'true' }, } path = 'Zabbix_Templates/Template_AWS_RDS.xml' files = glob.glob(path) for file in files: with open(file, 'r') as f: template = f.read() try: zapi.confimport('xml', template, rules) except ZabbixAPIException as e: print(e) return add_template_to_zabbix def main(): zapi = login_to_zabbix() Zabbix_Version = zapi.do_request('apiinfo.version') print(bgcolors().colors['OKGREEN'],"============================================================", bgcolors().colors['ENDC']) print(bgcolors().colors['OKGREEN'],'Zabbix Version:', Zabbix_Version['result'] + bgcolors().colors['ENDC']) print(bgcolors().colors['OKGREEN'],"============================================================", bgcolors().colors['ENDC']) # find needed group, template, host Needed_groups = ['AWS RDS'] Needed_IPs = get_rds_hosts_from_assum_role() Needed_Templates = ['Template_AWS_RDS'] Get_Templates = zapi.template.get(search={"name": Needed_Templates}) for template in Get_Templates: Needed_Template = template['name'] for Needed_group in Needed_groups: Get_Groups = zapi.hostgroup.get(search={"name": Needed_group}) if (len(Get_Groups)) is not 0: # check host in provided group for Needed_IP in Needed_IPs: Get_Hosts = zapi.host.get(search={"name": Needed_IP}) if (len(Get_Hosts)) is 0: #for h in Get_Hosts: add_host_to_zabbix(Needed_IP, Needed_group, Needed_Template) print('Added ', Needed_IP, 'host to zabbix') else: #pass print ('The', Needed_IP, 'already exist') else: add_group_to_zabbix(Needed_group) print ('Added the', Needed_group, 'group to zabbix') # find needed template for Needed_Template in Needed_Templates: Get_Templates = zapi.hostgroup.get(filter={"name": Needed_Templates}) if (len(Get_Templates)) is 0: add_template_to_zabbix() print('Added', Needed_Template, 'template to zabbix') else: pass for Needed_IP in Needed_IPs: Get_Hosts = zapi.host.get(filter={"name": Needed_IP}, output=['hostid', 'host', 'name', 'status']) if (len(Get_Hosts)) is 0: add_host_to_zabbix(Needed_IP, Needed_group, Needed_Template) print('Added ', Needed_IP, 'host to zabbix') else: pass print(bgcolors().colors['OKGREEN'], "============================================================", bgcolors().colors['ENDC']) print(bgcolors().colors['OKGREEN'], "==========================FINISHED==========================", bgcolors().colors['ENDC']) print(bgcolors().colors['OKGREEN'], "============================================================", bgcolors().colors['ENDC']) if __name__ == '__main__': main()
В теле скрипта нужно изменить некоторые переменные. Я не буду рассказывать что нужно менять, думаю и так очевидно, если нет — то можете написать в комментариях и я помогу с этим.
Создаем темплейт:
# mkdir Zabbix_Templates
Открываем файл:
# vim Zabbix_Templates/Template_AWS_RDS.xml
И вставляем в него:
<?xml version="1.0" encoding="UTF-8"?> <zabbix_export> <version>2.0</version> <date>2017-08-18T17:25:33Z</date> <groups> <group> <name>AWS RDS</name> </group> <group> <name>Templates</name> </group> </groups> <templates> <template> <template>Template_AWS_RDS</template> <name>Template_AWS_RDS</name> <description>AWS RDS monitoring</description> <groups> <group> <name>AWS RDS</name> </group> <group> <name>Templates</name> </group> </groups> <applications> <application> <name>RDS</name> </application> </applications> <items> <item> <name>RDS::BinLogDiskUsage</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.BinLogDiskUsage.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The amount of disk space occupied by binary logs on the master. Applies to MySQL read replicas.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::CPUUtilization</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.CPUUtilization.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>%</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The percentage of CPU utilization.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::DatabaseConnections</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.DatabaseConnections.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units/> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The number of database connections in use.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::DiskQueueDepth</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.DiskQueueDepth.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units/> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The number of outstanding IOs (read/write requests) waiting to access the disk.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::FreeableMemory</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.FreeableMemory.Minimum</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The amount of available random access memory.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::FreeStorageSpace</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.FreeStorageSpace.Minimum</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The amount of available storage space.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::NetworkReceiveThroughput</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.NetworkReceiveThroughput.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B/s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The incoming (Receive) network traffic on the DB instance, including both customer database traffic and Amazon RDS traffic used for monitoring and replication.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::NetworkTransmitThroughput</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.NetworkTransmitThroughput.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B/s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The outgoing (Transmit) network traffic on the DB instance, including both customer database traffic and Amazon RDS traffic used for monitoring and replication.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::ReadIOPS</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.ReadIOPS.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>ops/s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The average number of disk I/O operations per second.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::ReadLatency</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.ReadLatency.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The average amount of time taken per disk I/O operation.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::ReadThroughput</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.ReadThroughput.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B/s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The average number of bytes read from disk per second.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::ReplicaLag</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.ReplicaLag.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The amount of time a Read Replica DB Instance lags behind the source DB Instance. Applies to MySQL read replicas. The ReplicaLag metric reports the value of the Seconds_Behind_Master field of the MySQL SHOW SLAVE STATUS command.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::SwapUsage</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.SwapUsage.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The amount of swap space used on the DB Instance.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::WriteIOPS</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.WriteIOPS.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>ops/s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The average number of disk I/O operations per second.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::WriteLatency</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.WriteLatency.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The average amount of time taken per disk I/O operation.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> <item> <name>RDS::WriteThroughput</name> <type>2</type> <snmp_community/> <multiplier>0</multiplier> <snmp_oid/> <key>RDS.WriteThroughput.Average</key> <delay>0</delay> <history>15</history> <trends>365</trends> <status>0</status> <value_type>0</value_type> <allowed_hosts/> <units>B/s</units> <delta>0</delta> <snmpv3_contextname/> <snmpv3_securityname/> <snmpv3_securitylevel>0</snmpv3_securitylevel> <snmpv3_authprotocol>0</snmpv3_authprotocol> <snmpv3_authpassphrase/> <snmpv3_privprotocol>0</snmpv3_privprotocol> <snmpv3_privpassphrase/> <formula>1</formula> <delay_flex/> <params/> <ipmi_sensor/> <data_type>0</data_type> <authtype>0</authtype> <username/> <password/> <publickey/> <privatekey/> <port/> <description>The average number of bytes written to disk per second.</description> <inventory_link>0</inventory_link> <applications> <application> <name>RDS</name> </application> </applications> <valuemap/> <logtimefmt/> </item> </items> <discovery_rules/> <macros> <macro> <macro>{$TH_P2_FREE_STORAGE}</macro> <value>1G</value> </macro> <macro> <macro>{$TH_P2_LATENCY}</macro> <value>0.2</value> </macro> <macro> <macro>{$TH_P3_CPU_USAGE}</macro> <value>90</value> </macro> <macro> <macro>{$TH_P3_FREE_STORAGE}</macro> <value>2G</value> </macro> <macro> <macro>{$TH_P3_LATENCY}</macro> <value>0.1</value> </macro> <macro> <macro>{$TH_P4_CPU_USAGE}</macro> <value>85</value> </macro> </macros> <templates/> <screens> <screen> <name>RDS::Statistics</name> <hsize>2</hsize> <vsize>3</vsize> <screen_items> <screen_item> <resourcetype>0</resourcetype> <width>500</width> <height>100</height> <x>0</x> <y>0</y> <colspan>1</colspan> <rowspan>1</rowspan> <elements>0</elements> <valign>1</valign> <halign>2</halign> <style>0</style> <url/> <dynamic>0</dynamic> <sort_triggers>0</sort_triggers> <resource> <name>RDS::DB Connections</name> <host>Template_AWS_RDS</host> </resource> <max_columns>3</max_columns> <application/> </screen_item> <screen_item> <resourcetype>0</resourcetype> <width>500</width> <height>100</height> <x>1</x> <y>0</y> <colspan>1</colspan> <rowspan>1</rowspan> <elements>0</elements> <valign>1</valign> <halign>1</halign> <style>0</style> <url/> <dynamic>0</dynamic> <sort_triggers>0</sort_triggers> <resource> <name>RDS::Latency</name> <host>Template_AWS_RDS</host> </resource> <max_columns>3</max_columns> <application/> </screen_item> <screen_item> <resourcetype>0</resourcetype> <width>500</width> <height>100</height> <x>0</x> <y>1</y> <colspan>1</colspan> <rowspan>1</rowspan> <elements>0</elements> <valign>1</valign> <halign>2</halign> <style>0</style> <url/> <dynamic>0</dynamic> <sort_triggers>0</sort_triggers> <resource> <name>RDS::Storage Stats</name> <host>Template_AWS_RDS</host> </resource> <max_columns>3</max_columns> <application/> </screen_item> <screen_item> <resourcetype>0</resourcetype> <width>500</width> <height>100</height> <x>1</x> <y>1</y> <colspan>1</colspan> <rowspan>1</rowspan> <elements>0</elements> <valign>1</valign> <halign>1</halign> <style>0</style> <url/> <dynamic>0</dynamic> <sort_triggers>0</sort_triggers> <resource> <name>RDS::System Stats</name> <host>Template_AWS_RDS</host> </resource> <max_columns>3</max_columns> <application/> </screen_item> <screen_item> <resourcetype>0</resourcetype> <width>500</width> <height>100</height> <x>0</x> <y>2</y> <colspan>1</colspan> <rowspan>1</rowspan> <elements>0</elements> <valign>1</valign> <halign>2</halign> <style>0</style> <url/> <dynamic>0</dynamic> <sort_triggers>0</sort_triggers> <resource> <name>RDS::Network Stats</name> <host>Template_AWS_RDS</host> </resource> <max_columns>3</max_columns> <application/> </screen_item> <screen_item> <resourcetype>0</resourcetype> <width>500</width> <height>100</height> <x>1</x> <y>2</y> <colspan>1</colspan> <rowspan>1</rowspan> <elements>0</elements> <valign>1</valign> <halign>1</halign> <style>0</style> <url/> <dynamic>0</dynamic> <sort_triggers>0</sort_triggers> <resource> <name>RDS::IO Stats</name> <host>Template_AWS_RDS</host> </resource> <max_columns>3</max_columns> <application/> </screen_item> </screen_items> </screen> </screens> </template> </templates> <triggers> <trigger> <expression>{Template_AWS_RDS:RDS.CPUUtilization.Average.min(#3)}>{$TH_P4_CPU_USAGE} and {Template_AWS_RDS:RDS.CPUUtilization.Average.max(#3)}<{$TH_P3_CPU_USAGE}</expression> <name>RDS::High CPU Usage (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>2</priority> <description/> <type>0</type> <dependencies/> </trigger> <trigger> <expression>{Template_AWS_RDS:RDS.CPUUtilization.Average.min(#3)}>{$TH_P3_CPU_USAGE}</expression> <name>RDS::High CPU Usage (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>3</priority> <description/> <type>0</type> <dependencies/> </trigger> <trigger> <expression>{Template_AWS_RDS:RDS.ReadLatency.Average.last()}>{$TH_P3_LATENCY} and {Template_AWS_RDS:RDS.ReadLatency.Average.last()}<{$TH_P2_LATENCY}</expression> <name>RDS::High Read Latency (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>3</priority> <description/> <type>0</type> <dependencies/> </trigger> <trigger> <expression>{Template_AWS_RDS:RDS.ReadLatency.Average.last()}>{$TH_P2_LATENCY}</expression> <name>RDS::High Read Latency (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>4</priority> <description/> <type>0</type> <dependencies/> </trigger> <trigger> <expression>{Template_AWS_RDS:RDS.WriteLatency.Average.last()}>{$TH_P2_LATENCY}</expression> <name>RDS::High Write Latency (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>4</priority> <description/> <type>0</type> <dependencies/> </trigger> <trigger> <expression>{Template_AWS_RDS:RDS.WriteLatency.Average.last()}>{$TH_P3_LATENCY} and {Template_AWS_RDS:RDS.WriteLatency.Average.last()}<{$TH_P2_LATENCY}</expression> <name>RDS::High Write Latency (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>3</priority> <description/> <type>0</type> <dependencies/> </trigger> <trigger> <expression>{Template_AWS_RDS:RDS.FreeStorageSpace.Minimum.last()}<{$TH_P3_FREE_STORAGE} and {Template_AWS_RDS:RDS.FreeStorageSpace.Minimum.last()}>{$TH_P2_FREE_STORAGE}</expression> <name>RDS::Low free storage space (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>3</priority> <description/> <type>0</type> <dependencies/> </trigger> <trigger> <expression>{Template_AWS_RDS:RDS.FreeStorageSpace.Minimum.last()}<{$TH_P2_FREE_STORAGE}</expression> <name>RDS::Low free storage space (LV={ITEM.VALUE})</name> <url/> <status>0</status> <priority>4</priority> <description/> <type>0</type> <dependencies/> </trigger> </triggers> <graphs> <graph> <name>RDS::DB Connections</name> <width>800</width> <height>200</height> <yaxismin>0.0000</yaxismin> <yaxismax>100.0000</yaxismax> <show_work_period>1</show_work_period> <show_triggers>1</show_triggers> <type>0</type> <show_legend>1</show_legend> <show_3d>0</show_3d> <percent_left>0.0000</percent_left> <percent_right>0.0000</percent_right> <ymin_type_1>1</ymin_type_1> <ymax_type_1>0</ymax_type_1> <ymin_item_1>0</ymin_item_1> <ymax_item_1>0</ymax_item_1> <graph_items> <graph_item> <sortorder>0</sortorder> <drawtype>5</drawtype> <color>00C800</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.DatabaseConnections.Average</key> </item> </graph_item> </graph_items> </graph> <graph> <name>RDS::IO Stats</name> <width>800</width> <height>200</height> <yaxismin>0.0000</yaxismin> <yaxismax>100.0000</yaxismax> <show_work_period>1</show_work_period> <show_triggers>1</show_triggers> <type>0</type> <show_legend>1</show_legend> <show_3d>0</show_3d> <percent_left>0.0000</percent_left> <percent_right>0.0000</percent_right> <ymin_type_1>1</ymin_type_1> <ymax_type_1>0</ymax_type_1> <ymin_item_1>0</ymin_item_1> <ymax_item_1>0</ymax_item_1> <graph_items> <graph_item> <sortorder>0</sortorder> <drawtype>5</drawtype> <color>00CC00</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.ReadIOPS.Average</key> </item> </graph_item> <graph_item> <sortorder>1</sortorder> <drawtype>5</drawtype> <color>DDDD00</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.WriteIOPS.Average</key> </item> </graph_item> <graph_item> <sortorder>2</sortorder> <drawtype>0</drawtype> <color>CC0000</color> <yaxisside>0</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.DiskQueueDepth.Average</key> </item> </graph_item> </graph_items> </graph> <graph> <name>RDS::Latency</name> <width>800</width> <height>200</height> <yaxismin>0.0000</yaxismin> <yaxismax>100.0000</yaxismax> <show_work_period>1</show_work_period> <show_triggers>1</show_triggers> <type>0</type> <show_legend>1</show_legend> <show_3d>0</show_3d> <percent_left>0.0000</percent_left> <percent_right>0.0000</percent_right> <ymin_type_1>1</ymin_type_1> <ymax_type_1>0</ymax_type_1> <ymin_item_1>0</ymin_item_1> <ymax_item_1>0</ymax_item_1> <graph_items> <graph_item> <sortorder>0</sortorder> <drawtype>5</drawtype> <color>00CC00</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.ReadThroughput.Average</key> </item> </graph_item> <graph_item> <sortorder>1</sortorder> <drawtype>5</drawtype> <color>DDDD00</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.WriteThroughput.Average</key> </item> </graph_item> <graph_item> <sortorder>2</sortorder> <drawtype>0</drawtype> <color>0000DD</color> <yaxisside>0</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.ReadLatency.Average</key> </item> </graph_item> <graph_item> <sortorder>3</sortorder> <drawtype>0</drawtype> <color>DD0000</color> <yaxisside>0</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.WriteLatency.Average</key> </item> </graph_item> </graph_items> </graph> <graph> <name>RDS::Network Stats</name> <width>800</width> <height>200</height> <yaxismin>0.0000</yaxismin> <yaxismax>100.0000</yaxismax> <show_work_period>1</show_work_period> <show_triggers>1</show_triggers> <type>0</type> <show_legend>1</show_legend> <show_3d>0</show_3d> <percent_left>0.0000</percent_left> <percent_right>0.0000</percent_right> <ymin_type_1>1</ymin_type_1> <ymax_type_1>0</ymax_type_1> <ymin_item_1>0</ymin_item_1> <ymax_item_1>0</ymax_item_1> <graph_items> <graph_item> <sortorder>0</sortorder> <drawtype>5</drawtype> <color>0000DD</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.NetworkReceiveThroughput.Average</key> </item> </graph_item> <graph_item> <sortorder>1</sortorder> <drawtype>5</drawtype> <color>00CC00</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.NetworkTransmitThroughput.Average</key> </item> </graph_item> </graph_items> </graph> <graph> <name>RDS::Storage Stats</name> <width>800</width> <height>200</height> <yaxismin>0.0000</yaxismin> <yaxismax>100.0000</yaxismax> <show_work_period>1</show_work_period> <show_triggers>1</show_triggers> <type>0</type> <show_legend>1</show_legend> <show_3d>0</show_3d> <percent_left>0.0000</percent_left> <percent_right>0.0000</percent_right> <ymin_type_1>1</ymin_type_1> <ymax_type_1>0</ymax_type_1> <ymin_item_1>0</ymin_item_1> <ymax_item_1>0</ymax_item_1> <graph_items> <graph_item> <sortorder>0</sortorder> <drawtype>5</drawtype> <color>0000DD</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.BinLogDiskUsage.Average</key> </item> </graph_item> <graph_item> <sortorder>1</sortorder> <drawtype>5</drawtype> <color>00CC00</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.FreeStorageSpace.Minimum</key> </item> </graph_item> </graph_items> </graph> <graph> <name>RDS::System Stats</name> <width>800</width> <height>200</height> <yaxismin>0.0000</yaxismin> <yaxismax>100.0000</yaxismax> <show_work_period>1</show_work_period> <show_triggers>1</show_triggers> <type>0</type> <show_legend>1</show_legend> <show_3d>0</show_3d> <percent_left>0.0000</percent_left> <percent_right>0.0000</percent_right> <ymin_type_1>1</ymin_type_1> <ymax_type_1>0</ymax_type_1> <ymin_item_1>0</ymin_item_1> <ymax_item_1>0</ymax_item_1> <graph_items> <graph_item> <sortorder>0</sortorder> <drawtype>5</drawtype> <color>0000EE</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.SwapUsage.Average</key> </item> </graph_item> <graph_item> <sortorder>1</sortorder> <drawtype>5</drawtype> <color>00C800</color> <yaxisside>1</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.FreeableMemory.Minimum</key> </item> </graph_item> <graph_item> <sortorder>2</sortorder> <drawtype>2</drawtype> <color>EE0000</color> <yaxisside>0</yaxisside> <calc_fnc>7</calc_fnc> <type>0</type> <item> <host>Template_AWS_RDS</host> <key>RDS.CPUUtilization.Average</key> </item> </graph_item> </graph_items> </graph> </graphs> </zabbix_export>
Запускаем скрипт:
# python3 Add_Hosts_from_AWS_RDS_to_Zabbix.py
PS: Не забываем ставить зависимости через pip3.
Вот и все, статья «Добавление RDS хостов в zabbix c использованием boto3 + python3» завершена.