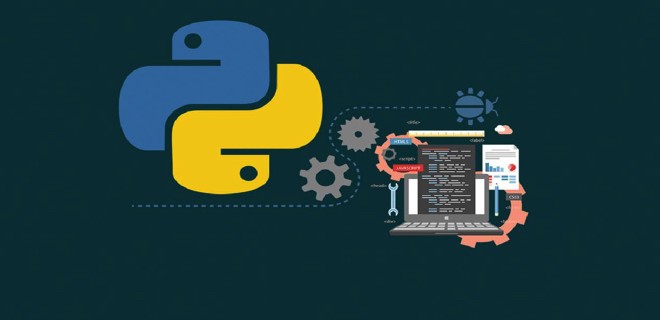
Генерация документации для Terraform с Python в Unix/Linux
Я уже напротяжении 6-8 месяцев, пишу терраформ-модули. Сначала это были модули для AWS. Сейчас для Google Cloud Platform (GCP). Планирую в этом году начать писать и для Azure (но об этом позже). Все модули я публикую на сайт + пушу все в github для общего использования. Меня напрягает однотипная, не оптимизированная задача, по этому — я решил сварганить «на коленке» скрипт.
Я придержуюсь единого стандарта для своих написанных Terraform модулей, например мой модуль имеет вид:
┌(captain@Macbook)─(✓)─(02:52 PM Fri Jul 06) └─(~/Projects/terraform/google_cloud_platform/modules/compute_instance_template)─(4 files, 48b)─> ll /Users/captain/Projects/terraform/google_cloud_platform/modules/compute_instance_template total 32 -rw-r--r--@ 1 captain staff 3020 Jul 6 10:42 compute_instance_template.tf -rw-r--r--@ 1 captain staff 309 Jul 6 10:42 output.tf drwxr-xr-x 3 captain staff 96 Jun 29 16:25 scripts -rw-r--r--@ 1 captain staff 8132 Jul 6 10:42 variables.tf ┌(captain@Macbook)─(✓)─(02:52 PM Fri Jul 06) └─(~/Projects/terraform/google_cloud_platform/modules/compute_instance_template)─(4 files, 48b)─>
Где:
- /Users/captain/Projects/terraform/google_cloud_platform/modules/compute_instance_template — Собственно путь где лежат все необходимые файлы для модуля.
- variables.tf — Файл со всеми переменными.
Файл с переменными имеет вид:
variable "name" { description = "A unique name for the resource, required by GCE. Changing this forces a new resource to be created." default = "TEST" } variable "region" { description = "An instance template is a global resource that is not bound to a zone or a region. However, you can still specify some regional resources in an instance template, which restricts the template to the region where that resource resides. For example, a custom subnetwork resource is tied to a specific region. Defaults to the region of the Provider if no value is given." default = "" } variable "project" { description = "The ID of the project in which the resource belongs. If it is not provided, the provider project is used." default = "" } variable "environment" { description = "Environment for service" default = "STAGE" } variable "orchestration" { description = "Type of orchestration" default = "Terraform" }
Это не полный файл, а только часть его. Но структура должна иметь именно такой вид! Иначе — все пропало, шеф!
Я на скорую руку, написал питон-скрипт для генерации доки:
$ cat generate_docs_for_terraform.py
Содержание скрипта:
#!/usr/bin/env python3 # -*- coding: utf-8 -*- import argparse import time import os import os.path class Bgcolors: def __init__(self): self.get = { 'HEADER': '\033[95m', 'OKBLUE': '\033[94m', 'OKGREEN': '\033[92m', 'WARNING': '\033[93m', 'FAIL': '\033[91m', 'ENDC': '\033[0m', 'BOLD': '\033[1m', 'UNDERLINE': '\033[4m' } def generate_docs_for_terraform(dir): if dir is not None: tf_file = dir + '/' + 'variables.tf' if os.path.isfile(tf_file) is False: print(Bgcolors().get['FAIL'], 'File not exist! Check PATH to module!', Bgcolors().get['ENDC']) exit(0) file_out = 'OUTPUT.txt' if os.path.isfile(file_out): os.remove(file_out) i = 0 j = 1 e = 2 step = 5 lines = open(tf_file).read().splitlines() while len(lines) >= e: variable = lines[i].split('"')[1] description = lines[j].split("=")[1] default = lines[e] line = '- `%s` -%s (`%s`)' % (variable, description, default) # print('- `%s` -%s (`%s`)' % (variable, description, default)) try: f = open(file_out, 'a') f.write(str(line + '\n')) f.close() except ValueError: print('I cant write to [%s] file' % file_out) print(ValueError) i += step j += step e += step else: print(Bgcolors().get['FAIL'], 'Please set/add [--dir]', Bgcolors().get['ENDC']) print(Bgcolors().get['OKGREEN'], 'For help, use: script_name.py -h', Bgcolors().get['ENDC']) exit(0) return generate_docs_for_terraform def main(): start__time = time.time() parser = argparse.ArgumentParser(prog='python3 script_name.py -h', usage='python3 script_name.py {ARGS}', add_help=True, prefix_chars='--/', epilog='''created by Vitalii Natarov''') parser.add_argument('--version', action='version', version='v1.0.0') parser.add_argument('--d', '--dir', dest='directory', help='Set directory where module exist', default=None, metavar='folder') results = parser.parse_args() # directory = results.directory generate_docs_for_terraform(directory) end__time = round(time.time() - start__time, 2) print("--- %s seconds ---" % end__time) print( Bgcolors().get['OKGREEN'], "============================================================", Bgcolors().get['ENDC']) print( Bgcolors().get['OKGREEN'], "==========================FINISHED==========================", Bgcolors().get['ENDC']) print( Bgcolors().get['OKGREEN'], "============================================================", Bgcolors().get['ENDC']) if __name__ == '__main__': main()
Для запуска, служит команда:
$ python3 generate_docs_for_terraform.py --dir="/Users/captain/Projects/terraform/google_cloud_platform/modules/compute_instance"
В директории где лежит скрипт, будет создан «OUTPUT.txt» файл, который будет иметь вид:
- `name` - "A unique name for the resource, required by GCE. Changing this forces a new resource to be created." (` default = "TEST"`) - `zone` - "The zone that the machine should be created in" (` default = "us-east1-b" `) - `environment` - "Environment for service" (` default = "STAGE"`) - `orchestration` - "Type of orchestration" (` default = "Terraform"`)
На скорую руку — солюшен зашел.
Хочу отметить то, что все мои terraform модули находятся:
$ git clone https://github.com/SebastianUA/terraform.git
А на этом, у меня пока все. Статья «Генерация документации для Terraform с Python в Unix/Linux» завершена.